Activate Texture toggle only when button is close
Quote from Goldenknighttim on July 23, 2014, 5:03 pmHey guys,
I'm working on my first multi-function script, so I'm not very familiar with common logic problems. Basically, around this function, the button is following the player around. I'm trying to make it so that if the button is pressed while it is 64 units or less away from a texture toggle, it increments the texture. I have a static variable called antiline that holds the closest texutretoggle. Here is one of the if statements i've tried:
if (antiline_detector() == true && button_pressed == true){
antiline = Entities.FindByClassnameNearest("env_texturetoggle",self.GetOrigin(),64);
local name = antiline.GetName();
ShowMessage(name);
self.ConnectOutput("OnPressed","DoEntFire(""+name+"","incrementtextureindex","",0,null,null)");
button_pressed = false;
}Another one I've tried is this:
if (antiline_detector() == true && button_pressed == true){
antiline = Entities.FindByClassnameNearest("env_texturetoggle",self.GetOrigin(),64);
self.ConnectOutput("OnPressed","EntFire(antiline,"incrementtextureindex","",0,null)");
button_pressed = false
}I've also tried a varient of the second one where the second perameter of the commectoutput function was seperated into it's own function. I doubt this will all work the way I want it to, and I'll be happy to run into those mistakes as I go, but my problem now is that when I press the button over the texture toggle entity, the console says the it fireing whenever I press the button, but the texture doesn't toggle. So, my problem is with the EntFire and DoEntFire functions. Does anyone see my problem with that?
Edit: I also tried this:
if (antiline_detector() == true && button_pressed == true){
antiline = Entities.FindByClassnameNearest("env_texturetoggle",self.GetOrigin(),64);
self.ConnectOutput("OnPressed","EntFireByHandle(antiline,"IncrementTextureIndex","",0,null,null)");
button_pressed = false
}
Hey guys,
I'm working on my first multi-function script, so I'm not very familiar with common logic problems. Basically, around this function, the button is following the player around. I'm trying to make it so that if the button is pressed while it is 64 units or less away from a texture toggle, it increments the texture. I have a static variable called antiline that holds the closest texutretoggle. Here is one of the if statements i've tried:
if (antiline_detector() == true && button_pressed == true){
antiline = Entities.FindByClassnameNearest("env_texturetoggle",self.GetOrigin(),64);
local name = antiline.GetName();
ShowMessage(name);
self.ConnectOutput("OnPressed","DoEntFire(""+name+"","incrementtextureindex","",0,null,null)");
button_pressed = false;
}
Another one I've tried is this:
if (antiline_detector() == true && button_pressed == true){
antiline = Entities.FindByClassnameNearest("env_texturetoggle",self.GetOrigin(),64);
self.ConnectOutput("OnPressed","EntFire(antiline,"incrementtextureindex","",0,null)");
button_pressed = false
}
I've also tried a varient of the second one where the second perameter of the commectoutput function was seperated into it's own function. I doubt this will all work the way I want it to, and I'll be happy to run into those mistakes as I go, but my problem now is that when I press the button over the texture toggle entity, the console says the it fireing whenever I press the button, but the texture doesn't toggle. So, my problem is with the EntFire and DoEntFire functions. Does anyone see my problem with that?
Edit: I also tried this:
if (antiline_detector() == true && button_pressed == true){
antiline = Entities.FindByClassnameNearest("env_texturetoggle",self.GetOrigin(),64);
self.ConnectOutput("OnPressed","EntFireByHandle(antiline,"IncrementTextureIndex","",0,null,null)");
button_pressed = false
}
Quote from FelixGriffin on July 23, 2014, 11:20 pmAre you sure you've actually found something with your FindByClassnameNearest? Compare the result against null to make sure before you do anything with it.
Since "antiline" is presumably a global variable, there's no need to use ConnectOutput. Just add a function like this...
- Code: Select all
function toggleTexture(){ EntFireByHandle(antiline,"IncrementTextureIndex","",0.0,null,null); }
...and an output like this on your button...
- Code: Select all
OnPressed !self RunScriptCode toggleTexture();
...then update "antiline" every time a new toggle is detected.
Since env_texturetoggles don't use their origin normally, I would use info_targets with a shared targetname ("@antline_toggle" or something like that) which call IncrementTextureIndex when their User1 input is fired. Then detect those by targetname and use FireUser1 instead of IncrementTextureIndex directly.
Are you sure you've actually found something with your FindByClassnameNearest? Compare the result against null to make sure before you do anything with it.
Since "antiline" is presumably a global variable, there's no need to use ConnectOutput. Just add a function like this...
- Code: Select all
function toggleTexture(){ EntFireByHandle(antiline,"IncrementTextureIndex","",0.0,null,null); }
...and an output like this on your button...
- Code: Select all
OnPressed !self RunScriptCode toggleTexture();
...then update "antiline" every time a new toggle is detected.
Since env_texturetoggles don't use their origin normally, I would use info_targets with a shared targetname ("@antline_toggle" or something like that) which call IncrementTextureIndex when their User1 input is fired. Then detect those by targetname and use FireUser1 instead of IncrementTextureIndex directly.
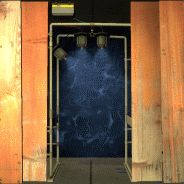
Quote from TeamSpen210 on July 23, 2014, 11:43 pmAlternately, you could just surround each texture_toggle with a trigger_multiple named @button_trig or something. (Put it in an instance with the toggle and a $replace variable, just ensure the indicator lights have an @ prefix.) When the button is pressed TouchTest the @button_trig, OnTouching IncrementTextureIndex. This way the player must be inside the trigger to be able to toggle the indicators. You might want to ToggleTest a logic_branch also so you can acually detect the setting of the indicators. (ProxyRelay the OnTrue and OnFalse outputs, then you can just place an instance to have it all setup for you.
Alternately, you could just surround each texture_toggle with a trigger_multiple named @button_trig or something. (Put it in an instance with the toggle and a $replace variable, just ensure the indicator lights have an @ prefix.) When the button is pressed TouchTest the @button_trig, OnTouching IncrementTextureIndex. This way the player must be inside the trigger to be able to toggle the indicators. You might want to ToggleTest a logic_branch also so you can acually detect the setting of the indicators. (ProxyRelay the OnTrue and OnFalse outputs, then you can just place an instance to have it all setup for you.
[spoiler]- BEE2 Addons | (BEE2)
- Hammer Addons
Maps:
- Crushed Gel
- Gel is Not Always Helpful[/spoiler]
Quote from Goldenknighttim on July 24, 2014, 6:29 amThanks guys, I would definitely use TeamSpen's idea if I was more focused on the end goal, but My reason for doing this is to experiment with scripting. I would prefer for my experiment to do as little with hammer as possible. I don't mind putting targets around the lights using the same name, however I do know that GetName() in the first listed if statement is actually getting the name, so I'm not sure that will be necessary. I'll try it on the train ride to work this morning and let you know how it goes. I was hoping I was missing something obvious with the connect output function.
Thanks guys, I would definitely use TeamSpen's idea if I was more focused on the end goal, but My reason for doing this is to experiment with scripting. I would prefer for my experiment to do as little with hammer as possible. I don't mind putting targets around the lights using the same name, however I do know that GetName() in the first listed if statement is actually getting the name, so I'm not sure that will be necessary. I'll try it on the train ride to work this morning and let you know how it goes. I was hoping I was missing something obvious with the connect output function.
Quote from Goldenknighttim on July 24, 2014, 8:04 amI tried using the DoEntFire with an info_target with the same name as the texture toggle. That didn't change anything, but I have seen much evidence that the texture toggle has an origin. The picture I attached shows what happens in the console when I press the button, if you want to have a look there. I'm not sure of this, but it looks like the button is firing the output twice in a row. I wonder if it is toggling the texture, but twice, and too fast to see it. I can't see why it would do that, but I'll look more into that.
I tried using the DoEntFire with an info_target with the same name as the texture toggle. That didn't change anything, but I have seen much evidence that the texture toggle has an origin. The picture I attached shows what happens in the console when I press the button, if you want to have a look there. I'm not sure of this, but it looks like the button is firing the output twice in a row. I wonder if it is toggling the texture, but twice, and too fast to see it. I can't see why it would do that, but I'll look more into that.
Quote from FelixGriffin on July 24, 2014, 10:03 amCallScriptFunction has a few odd quirks. Use RunScriptCode instead, or (if it's just an EntFire) the desired output directly.
CallScriptFunction has a few odd quirks. Use RunScriptCode instead, or (if it's just an EntFire) the desired output directly.
Quote from Goldenknighttim on July 24, 2014, 10:11 amI didn't type CallScriptFunction anywhere, the ConnectOutput function must be using that. Is there a way to look at how these functions are defined so I can write my own that uses RunScriptCode instead of CallScriptFunction? I'm not sure how I can get it to happen "OnPressed" without using ConnectOutput.
I didn't type CallScriptFunction anywhere, the ConnectOutput function must be using that. Is there a way to look at how these functions are defined so I can write my own that uses RunScriptCode instead of CallScriptFunction? I'm not sure how I can get it to happen "OnPressed" without using ConnectOutput.
Quote from FelixGriffin on July 24, 2014, 10:49 pmDon't use ConnectOutput either. You're going to add a new copy of the output every time the button gets close, so the first time it will toggle once, the next time twice, and so on.
Don't use ConnectOutput either. You're going to add a new copy of the output every time the button gets close, so the first time it will toggle once, the next time twice, and so on.