Scripting Questions, FindByClassnameNearest()
Quote from Goldenknighttim on September 9, 2014, 9:05 amHey guys, I'm trying to write a script that can be run in multiple places in the same map, each time from within it's own instance. There are 2 entities in the instance that I want saved as variables. When I set the instance to not prefex or suffex the name, then I can easily store the entities to the name with FindByName(). This was great for testing, but to make this instance an easy plug-and-play instance, I cannot use this method. So what I tried next was:
- Code: Select all
laser_emitter <- Entities.FindByNameNearest("*Rev_Red_Laser",self.GetOrigin(), 32)
target_ent <- Entities.FindByNameNearest("*Rev_Red_Laser_end",self.GetOrigin(),32)I did this after setting the instance to set a prefix to the name again. When things weren't working as I expected, and I tested with printl(target_ent.GetName()), it gave me the name of the object running the script: "(prefix)-Rev_Red_Laser_start". Does a '*' at the beginning effect the entire string? I would think it would be for just the beginning. I next tried this:
- Code: Select all
laser_emitter <- FindByClassnameNearest("prop_dynamic", self.GetOrigin(), 32);
target_ent <- FindByClassnameNearest("info_target", self.GetOrigin(), 32);It however seems like portal doesn't recognize the FindByClassnameNearest() function because after I entered this, it started saying that main() does not exist. (main() is the first function called.) I don't understand why this is giving me so much trouble. Are these functions known to have issues? Is there anything I'm doing that would make these functions not work?
Hey guys, I'm trying to write a script that can be run in multiple places in the same map, each time from within it's own instance. There are 2 entities in the instance that I want saved as variables. When I set the instance to not prefex or suffex the name, then I can easily store the entities to the name with FindByName(). This was great for testing, but to make this instance an easy plug-and-play instance, I cannot use this method. So what I tried next was:
- Code: Select all
laser_emitter <- Entities.FindByNameNearest("*Rev_Red_Laser",self.GetOrigin(), 32)
target_ent <- Entities.FindByNameNearest("*Rev_Red_Laser_end",self.GetOrigin(),32)
I did this after setting the instance to set a prefix to the name again. When things weren't working as I expected, and I tested with printl(target_ent.GetName()), it gave me the name of the object running the script: "(prefix)-Rev_Red_Laser_start". Does a '*' at the beginning effect the entire string? I would think it would be for just the beginning. I next tried this:
- Code: Select all
laser_emitter <- FindByClassnameNearest("prop_dynamic", self.GetOrigin(), 32);
target_ent <- FindByClassnameNearest("info_target", self.GetOrigin(), 32);
It however seems like portal doesn't recognize the FindByClassnameNearest() function because after I entered this, it started saying that main() does not exist. (main() is the first function called.) I don't understand why this is giving me so much trouble. Are these functions known to have issues? Is there anything I'm doing that would make these functions not work?
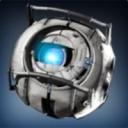
Quote from Gemarakup on September 9, 2014, 9:35 amI don't know. The only thing I know is that you can just use multiple names and scripts.
I don't know. The only thing I know is that you can just use multiple names and scripts.
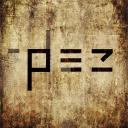
Quote from josepezdj on September 9, 2014, 9:36 amI just reminded one script I recently read which opens nearby (to the player) linked_doors. The function used is "FindByClassnameWithin()" though, but it might help you out to get your goal anyways:
open_nearby_portal_linked_doors.nut
[spoiler]
- Code: Select all
// Execute this script from the console to open nearby prop_portal_linked_doors.
// Intended to be used to traverse maps in order without noclipping to find the next linked map.ent <- null
if ( ent = Entities.FindByClassnameWithin( ent, "prop_linked_portal_door", player.GetOrigin(), 256 ) )
{
EntFire( ent.GetName(), "Open", "", 0.0, null );
}[/spoiler]
The usage of that function makes me think the rest ones are also actually functional....
I just reminded one script I recently read which opens nearby (to the player) linked_doors. The function used is "FindByClassnameWithin()" though, but it might help you out to get your goal anyways:
open_nearby_portal_linked_doors.nut
The usage of that function makes me think the rest ones are also actually functional....
Quote from Goldenknighttim on September 9, 2014, 9:38 amForgot the "Entities." I got it working now. Don't worry about the findbyclassnamenearest questions, but the glitch with the '*' still confuses me, so if you understand what was happening there, some clearification would still be nice.
Forgot the "Entities." I got it working now. Don't worry about the findbyclassnamenearest questions, but the glitch with the '*' still confuses me, so if you understand what was happening there, some clearification would still be nice.
Quote from FelixGriffin on September 9, 2014, 10:07 amAFAIK wildcard matching in Source is fairly weak: a star literally means something like "if it matches up to this point, say it was a valid match". So you can only use it meaningfully at the end of the name.
If you are having fixup difficulties, you can put the instance inside another instance, and set the inner func_instance's fixup mode to "none".
AFAIK wildcard matching in Source is fairly weak: a star literally means something like "if it matches up to this point, say it was a valid match". So you can only use it meaningfully at the end of the name.
If you are having fixup difficulties, you can put the instance inside another instance, and set the inner func_instance's fixup mode to "none".
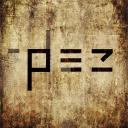
Quote from josepezdj on September 9, 2014, 10:27 amI also reminded that HMW wrote a script using the FindByClassnameNearest() function (I knew I had already seen it before :p):
sticky_panels.nut
[spoiler]
- Code: Select all
//
// hmw/sticky_panels.nut
// ________________________________________________________________________
//
// Script to attach entities to a model's attachment points by proximity,
// rather than by name.
//
// (Example: attach a func_brush surface tile to an animated panel.)
//
// When the model finishes its first animation (to set the bind pose),
// it will look for an entity of class find_class within search_radius
// or less from attachment attach_name, and send a "SetParent" signal,
// followed by "SetParentAttachmentMaintainOffset".
//
// Intended for use by the "entity scripts" attribute.
//
// Entities may modify the default values for find_class, attach_name
// and search_radius.
// ________________________________________________________________________find_class <- "func_brush";
attach_name <- "panel_attach";
search_radius <- 2;function attach_item()
{
local attachment = self.LookupAttachment(attach_name);
local search_origin = self.GetAttachmentOrigin(attachment);
attached_part <- Entities.FindByClassnameNearest(
find_class, search_origin, search_radius);if(attached_part != null) {
EntFireByHandle(attached_part, "SetParent",
self.GetName(), 0, null, null);
EntFireByHandle(attached_part, "SetParentAttachmentMaintainOffset",
attach_name, 0, null, null);
};
};EntFireByHandle(self, "AddOutput",
"OnAnimationDone !self:RunScriptCode:attach_item():0:1",
0, null, null);// vim:set shiftwidth=4 softtabstop=4 expandtab nowrap filetype=squirrel:
[/spoiler]
Maybe that one could help you out a bit more.I used the asterisk as wildcard for some I/O in Hammer in the past succesfully... however, you might be having an issue with "finding the nearest" while saying "all entities starting by Rev_Red_Laser_end" (which could be not meaning any), could that be?
I also reminded that HMW wrote a script using the FindByClassnameNearest() function (I knew I had already seen it before :p):
sticky_panels.nut
Maybe that one could help you out a bit more.
I used the asterisk as wildcard for some I/O in Hammer in the past succesfully... however, you might be having an issue with "finding the nearest" while saying "all entities starting by Rev_Red_Laser_end" (which could be not meaning any), could that be?
Quote from Goldenknighttim on September 9, 2014, 12:03 pmThanks for the help guys.
Thanks for the help guys.
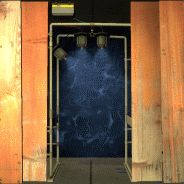
Quote from TeamSpen210 on September 9, 2014, 4:38 pmOne thing you could do instead is just self.GetName(), then do some string editing to find out what the user named your instance and then get a handle to the other entities.
One thing you could do instead is just self.GetName(), then do some string editing to find out what the user named your instance and then get a handle to the other entities.
[spoiler]- BEE2 Addons | (BEE2)
- Hammer Addons
Maps:
- Crushed Gel
- Gel is Not Always Helpful[/spoiler]
Quote from greykarel on May 2, 2015, 2:05 pmEDIT.
greykarel wrote:blah-blah-blah about some problem with a scriptI don't now whay was wrong before but today it works fine.
EDIT.
I don't now whay was wrong before but today it works fine.